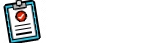
- 9.3.5. Class and Instance Variables
- -----------------------------------
- Generally speaking, instance variables are for data unique to each
- instance and class variables are for attributes and methods shared by
- all instances of the class:
- class Dog:
- kind = 'canine' # class variable shared by all instances
- def __init__(self, name):
- self.name = name # instance variable unique to each instance
- >>> d = Dog('Fido')
- >>> e = Dog('Buddy')
- >>> d.kind # shared by all dogs
- 'canine'
- >>> e.kind # shared by all dogs
- 'canine'
- >>> d.name # unique to d
- 'Fido'
- >>> e.name # unique to e
- 'Buddy'
- As discussed in A Word About Names and Objects, shared data can have
- possibly surprising effects with involving *mutable* objects such as
- lists and dictionaries. For example, the *tricks* list in the
- following code should not be used as a class variable because just a
- single list would be shared by all *Dog* instances:
- class Dog:
- tricks = [] # mistaken use of a class variable
- def __init__(self, name):
- self.name = name
- def add_trick(self, trick):
- self.tricks.append(trick)
- >>> d = Dog('Fido')
- >>> e = Dog('Buddy')
- >>> d.add_trick('roll over')
- >>> e.add_trick('play dead')
- >>> d.tricks # unexpectedly shared by all dogs
- ['roll over', 'play dead']
- Correct design of the class should use an instance variable instead:
- class Dog:
- def __init__(self, name):
- self.name = name
- self.tricks = [] # creates a new empty list for each dog
- def add_trick(self, trick):
- self.tricks.append(trick)
- >>> d = Dog('Fido')
- >>> e = Dog('Buddy')
- >>> d.add_trick('roll over')
- >>> e.add_trick('play dead')
- >>> d.tricks
- ['roll over']
- >>> e.tricks
- ['play dead']
Python software and documentation are licensed under the PSF License Agreement.
Starting with Python 3.8.6, examples, recipes, and other code in the documentation are dual licensed under the PSF License Agreement and the Zero-Clause BSD license.
Some software incorporated into Python is under different licenses. The licenses are listed with code falling under that license. See Licenses and Acknowledgements for Incorporated Software for an incomplete list of these licenses.
Python and it's documentation is:
Copyright © 2001-2022 Python Software Foundation. All rights reserved.
Copyright © 2000 BeOpen.com. All rights reserved.
Copyright © 1995-2000 Corporation for National Research Initiatives. All rights reserved.
Copyright © 1991-1995 Stichting Mathematisch Centrum. All rights reserved.
See History and License for complete license and permissions information:
https://docs.python.org/3/license.html#psf-license