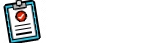
- 3.1. Objects, values and types
- ==============================
- *Objects* are Python's abstraction for data. All data in a Python
- program is represented by objects or by relations between objects. (In
- a sense, and in conformance to Von Neumann's model of a "stored
- program computer", code is also represented by objects.)
- Every object has an identity, a type and a value. An object's
- *identity* never changes once it has been created; you may think of it
- as the object's address in memory. The '"is"' operator compares the
- identity of two objects; the "id()" function returns an integer
- representing its identity.
- **CPython implementation detail:** For CPython, "id(x)" is the memory
- address where "x" is stored.
- An object's type determines the operations that the object supports
- (e.g., "does it have a length?") and also defines the possible values
- for objects of that type. The "type()" function returns an object's
- type (which is an object itself). Like its identity, an object's
- *type* is also unchangeable. [1]
- The *value* of some objects can change. Objects whose value can
- change are said to be *mutable*; objects whose value is unchangeable
- once they are created are called *immutable*. (The value of an
- immutable container object that contains a reference to a mutable
- object can change when the latter's value is changed; however the
- container is still considered immutable, because the collection of
- objects it contains cannot be changed. So, immutability is not
- strictly the same as having an unchangeable value, it is more subtle.)
- An object's mutability is determined by its type; for instance,
- numbers, strings and tuples are immutable, while dictionaries and
- lists are mutable.
- Objects are never explicitly destroyed; however, when they become
- unreachable they may be garbage-collected. An implementation is
- allowed to postpone garbage collection or omit it altogether --- it is
- a matter of implementation quality how garbage collection is
- implemented, as long as no objects are collected that are still
- reachable.
- **CPython implementation detail:** CPython currently uses a reference-
- counting scheme with (optional) delayed detection of cyclically linked
- garbage, which collects most objects as soon as they become
- unreachable, but is not guaranteed to collect garbage containing
- circular references. See the documentation of the "gc" module for
- information on controlling the collection of cyclic garbage. Other
- implementations act differently and CPython may change. Do not depend
- on immediate finalization of objects when they become unreachable (so
- you should always close files explicitly).
- Note that the use of the implementation's tracing or debugging
- facilities may keep objects alive that would normally be collectable.
- Also note that catching an exception with a '"try"..."except"'
- statement may keep objects alive.
- Some objects contain references to "external" resources such as open
- files or windows. It is understood that these resources are freed
- when the object is garbage-collected, but since garbage collection is
- not guaranteed to happen, such objects also provide an explicit way to
- release the external resource, usually a "close()" method. Programs
- are strongly recommended to explicitly close such objects. The
- '"try"..."finally"' statement and the '"with"' statement provide
- convenient ways to do this.
- Some objects contain references to other objects; these are called
- *containers*. Examples of containers are tuples, lists and
- dictionaries. The references are part of a container's value. In
- most cases, when we talk about the value of a container, we imply the
- values, not the identities of the contained objects; however, when we
- talk about the mutability of a container, only the identities of the
- immediately contained objects are implied. So, if an immutable
- container (like a tuple) contains a reference to a mutable object, its
- value changes if that mutable object is changed.
- Types affect almost all aspects of object behavior. Even the
- importance of object identity is affected in some sense: for immutable
- types, operations that compute new values may actually return a
- reference to any existing object with the same type and value, while
- for mutable objects this is not allowed. E.g., after "a = 1; b = 1",
- "a" and "b" may or may not refer to the same object with the value
- one, depending on the implementation, but after "c = []; d = []", "c"
- and "d" are guaranteed to refer to two different, unique, newly
- created empty lists. (Note that "c = d = []" assigns the same object
- to both "c" and "d".)
- Questions:
- What are objects in Python?
- What is an example of an object in Python?
- How do Python objects work?
- What is Python object-oriented programming?
- Is OOP in Python hard?
- Python Object Oriented Programming (OOP) - For Beginners
Python software and documentation are licensed under the PSF License Agreement.
Starting with Python 3.8.6, examples, recipes, and other code in the documentation are dual licensed under the PSF License Agreement and the Zero-Clause BSD license.
Some software incorporated into Python is under different licenses. The licenses are listed with code falling under that license. See Licenses and Acknowledgements for Incorporated Software for an incomplete list of these licenses.
Python and it's documentation is:
Copyright © 2001-2022 Python Software Foundation. All rights reserved.
Copyright © 2000 BeOpen.com. All rights reserved.
Copyright © 1995-2000 Corporation for National Research Initiatives. All rights reserved.
Copyright © 1991-1995 Stichting Mathematisch Centrum. All rights reserved.
See History and License for complete license and permissions information:
https://docs.python.org/3/license.html#psf-license