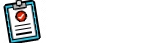
- The "argparse" module's support for command-line interfaces is built
- around an instance of "argparse.ArgumentParser". It is a container
- for argument specifications and has options that apply the parser as
- whole:
- parser = argparse.ArgumentParser(
- prog = 'ProgramName',
- description = 'What the program does',
- epilog = 'Text at the bottom of help')
- The "ArgumentParser.add_argument()" method attaches individual
- argument specifications to the parser. It supports positional
- arguments, options that accept values, and on/off flags:
- parser.add_argument('filename') # positional argument
- parser.add_argument('-c', '--count') # option that takes a value
- parser.add_argument('-v', '--verbose',
- action='store_true') # on/off flag
- The "ArgumentParser.parse_args()" method runs the parser and places
- the extracted data in a "argparse.Namespace" object:
- args = parser.parse_args()
- print(args.filename, args.count, args.verbose)
- Example
- =======
- The following code is a Python program that takes a list of integers
- and produces either the sum or the max:
- import argparse
- parser = argparse.ArgumentParser(description='Process some integers.')
- parser.add_argument('integers', metavar='N', type=int, nargs='+',
- help='an integer for the accumulator')
- parser.add_argument('--sum', dest='accumulate', action='store_const',
- const=sum, default=max,
- help='sum the integers (default: find the max)')
- args = parser.parse_args()
- print(args.accumulate(args.integers))
- Assuming the above Python code is saved into a file called "prog.py",
- it can be run at the command line and it provides useful help
- messages:
- $ python prog.py -h
- usage: prog.py [-h] [--sum] N [N ...]
- Process some integers.
- positional arguments:
- N an integer for the accumulator
- options:
- -h, --help show this help message and exit
- --sum sum the integers (default: find the max)
- When run with the appropriate arguments, it prints either the sum or
- the max of the command-line integers:
- $ python prog.py 1 2 3 4
- 4
- $ python prog.py 1 2 3 4 --sum
- 10
- If invalid arguments are passed in, an error will be displayed:
- $ python prog.py a b c
- usage: prog.py [-h] [--sum] N [N ...]
- prog.py: error: argument N: invalid int value: 'a'
- The following sections walk you through this example.
- Creating a parser
- -----------------
- The first step in using the "argparse" is creating an "ArgumentParser"
- object:
- >>> parser = argparse.ArgumentParser(description='Process some integers.')
- The "ArgumentParser" object will hold all the information necessary to
- parse the command line into Python data types.
- Adding arguments
- ----------------
- Filling an "ArgumentParser" with information about program arguments
- is done by making calls to the "add_argument()" method. Generally,
- these calls tell the "ArgumentParser" how to take the strings on the
- command line and turn them into objects. This information is stored
- and used when "parse_args()" is called. For example:
- >>> parser.add_argument('integers', metavar='N', type=int, nargs='+',
- ... help='an integer for the accumulator')
- >>> parser.add_argument('--sum', dest='accumulate', action='store_const',
- ... const=sum, default=max,
- ... help='sum the integers (default: find the max)')
- Later, calling "parse_args()" will return an object with two
- attributes, "integers" and "accumulate". The "integers" attribute
- will be a list of one or more integers, and the "accumulate" attribute
- will be either the "sum()" function, if "--sum" was specified at the
- command line, or the "max()" function if it was not.
- Parsing arguments
- -----------------
- "ArgumentParser" parses arguments through the "parse_args()" method.
- This will inspect the command line, convert each argument to the
- appropriate type and then invoke the appropriate action. In most
- cases, this means a simple "Namespace" object will be built up from
- attributes parsed out of the command line:
- >>> parser.parse_args(['--sum', '7', '-1', '42'])
- Namespace(accumulate=<built-in function sum>, integers=[7, -1, 42])
- In a script, "parse_args()" will typically be called with no
- arguments, and the "ArgumentParser" will automatically determine the
- command-line arguments from "sys.argv".
- ArgumentParser objects
- ======================
- class argparse.ArgumentParser(prog=None, usage=None, description=None, epilog=None, parents=[], formatter_class=argparse.HelpFormatter, prefix_chars='-', fromfile_prefix_chars=None, argument_default=None, conflict_handler='error', add_help=True, allow_abbrev=True, exit_on_error=True)
- Create a new "ArgumentParser" object. All parameters should be
- passed as keyword arguments. Each parameter has its own more
- detailed description below, but in short they are:
- * prog - The name of the program (default:
- "os.path.basename(sys.argv[0])")
- * usage - The string describing the program usage (default:
- generated from arguments added to parser)
- * description - Text to display before the argument help (by
- default, no text)
- * epilog - Text to display after the argument help (by default, no
- text)
- * parents - A list of "ArgumentParser" objects whose arguments
- should also be included
- * formatter_class - A class for customizing the help output
- * prefix_chars - The set of characters that prefix optional
- arguments (default: '-')
- * fromfile_prefix_chars - The set of characters that prefix files
- from which additional arguments should be read (default: "None")
- * argument_default - The global default value for arguments
- (default: "None")
- * conflict_handler - The strategy for resolving conflicting
- optionals (usually unnecessary)
- * add_help - Add a "-h/--help" option to the parser (default:
- "True")
- * allow_abbrev - Allows long options to be abbreviated if the
- abbreviation is unambiguous. (default: "True")
- * exit_on_error - Determines whether or not ArgumentParser exits
- with error info when an error occurs. (default: "True")
- Changed in version 3.5: *allow_abbrev* parameter was added.
- Changed in version 3.8: In previous versions, *allow_abbrev* also
- disabled grouping of short flags such as "-vv" to mean "-v -v".
- Changed in version 3.9: *exit_on_error* parameter was added.
- The following sections describe how each of these are used.
- prog
- ----
- By default, "ArgumentParser" objects use "sys.argv[0]" to determine
- how to display the name of the program in help messages. This default
- is almost always desirable because it will make the help messages
- match how the program was invoked on the command line. For example,
- consider a file named "myprogram.py" with the following code:
- import argparse
- parser = argparse.ArgumentParser()
- parser.add_argument('--foo', help='foo help')
- args = parser.parse_args()
- The help for this program will display "myprogram.py" as the program
- name (regardless of where the program was invoked from):
- $ python myprogram.py --help
- usage: myprogram.py [-h] [--foo FOO]
- options:
- -h, --help show this help message and exit
- --foo FOO foo help
- $ cd ..
- $ python subdir/myprogram.py --help
- usage: myprogram.py [-h] [--foo FOO]
- options:
- -h, --help show this help message and exit
- --foo FOO foo help
- To change this default behavior, another value can be supplied using
- the "prog=" argument to "ArgumentParser":
- >>> parser = argparse.ArgumentParser(prog='myprogram')
- >>> parser.print_help()
- usage: myprogram [-h]
- options:
- -h, --help show this help message and exit
- Note that the program name, whether determined from "sys.argv[0]" or
- from the "prog=" argument, is available to help messages using the
- "%(prog)s" format specifier.
- >>> parser = argparse.ArgumentParser(prog='myprogram')
- >>> parser.add_argument('--foo', help='foo of the %(prog)s program')
- >>> parser.print_help()
- usage: myprogram [-h] [--foo FOO]
- options:
- -h, --help show this help message and exit
- --foo FOO foo of the myprogram program
- usage
- -----
- By default, "ArgumentParser" calculates the usage message from the
- arguments it contains:
- >>> parser = argparse.ArgumentParser(prog='PROG')
- >>> parser.add_argument('--foo', nargs='?', help='foo help')
- >>> parser.add_argument('bar', nargs='+', help='bar help')
- >>> parser.print_help()
- usage: PROG [-h] [--foo [FOO]] bar [bar ...]
- positional arguments:
- bar bar help
- options:
- -h, --help show this help message and exit
- --foo [FOO] foo help
- The default message can be overridden with the "usage=" keyword
- argument:
- >>> parser = argparse.ArgumentParser(prog='PROG', usage='%(prog)s [options]')
- >>> parser.add_argument('--foo', nargs='?', help='foo help')
- >>> parser.add_argument('bar', nargs='+', help='bar help')
- >>> parser.print_help()
- usage: PROG [options]
- positional arguments:
- bar bar help
- options:
- -h, --help show this help message and exit
- --foo [FOO] foo help
- The "%(prog)s" format specifier is available to fill in the program
- name in your usage messages.
- Video tutorials:
- Argparse Basics - How I run my scripts via the Command Line
- Python argparse tutorial (beginner - intermediate)
- Understanding Python: Argparse CLI
- [inline]
Python software and documentation are licensed under the PSF License Agreement.
Starting with Python 3.8.6, examples, recipes, and other code in the documentation are dual licensed under the PSF License Agreement and the Zero-Clause BSD license.
Some software incorporated into Python is under different licenses. The licenses are listed with code falling under that license. See Licenses and Acknowledgements for Incorporated Software for an incomplete list of these licenses.
Python and it's documentation is:
Copyright © 2001-2022 Python Software Foundation. All rights reserved.
Copyright © 2000 BeOpen.com. All rights reserved.
Copyright © 1995-2000 Corporation for National Research Initiatives. All rights reserved.
Copyright © 1991-1995 Stichting Mathematisch Centrum. All rights reserved.
See History and License for complete license and permissions information:
https://docs.python.org/3/license.html#psf-license