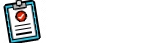
- To prevent SQL injection in Python, you can use the "parameterized queries" feature in Python's database API. This allows you to specify placeholders in your SQL query, and then provide the actual values as separate parameters. This ensures that the values are treated as data and not part of the SQL query, which protects against SQL injection attacks.
- Here is an example of how to use parameterized queries in Python to prevent SQL injection:
- Copy code
- # Import the database API module
- import sqlite3
- # Connect to the database
- db = sqlite3.connect("database.db")
- # Create a cursor object
- cursor = db.cursor()
- # Define the SQL query with placeholders
- query = "SELECT * FROM users WHERE username = ? AND password = ?"
- # Provide the actual values as separate parameters
- cursor.execute(query, ("johnsmith", "password123"))
- # Fetch the results
- results = cursor.fetchall()
- # Loop through the results and print them
- for row in results:
- print(row)
- # Close the cursor and the database connection
- cursor.close()
- db.close()
- In this example, the values for the username and password are provided as separate parameters, rather than being included directly in the SQL query. This protects against SQL injection attacks, as the values are treated as data.
Python software and documentation are licensed under the PSF License Agreement.
Starting with Python 3.8.6, examples, recipes, and other code in the documentation are dual licensed under the PSF License Agreement and the Zero-Clause BSD license.
Some software incorporated into Python is under different licenses. The licenses are listed with code falling under that license. See Licenses and Acknowledgements for Incorporated Software for an incomplete list of these licenses.
Python and it's documentation is:
Copyright © 2001-2022 Python Software Foundation. All rights reserved.
Copyright © 2000 BeOpen.com. All rights reserved.
Copyright © 1995-2000 Corporation for National Research Initiatives. All rights reserved.
Copyright © 1991-1995 Stichting Mathematisch Centrum. All rights reserved.
See History and License for complete license and permissions information:
https://docs.python.org/3/license.html#psf-license