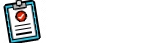
- <!DOCTYPE html>
- <html>
- <head>
- <title>Employee Search</title>
- <style>
- table {
- width: 100%;
- border-collapse: collapse;
- }
- th, td {
- border: 1px solid black;
- padding: 8px;
- text-align: left;
- }
- th {
- background-color: #f2f2f2;
- }
- </style>
- </head>
- <body>
- <h2>Employee Search</h2>
- <form method="GET" action="<?php echo $_SERVER['PHP_SELF']; ?>">
- <label for="emp_no">Enter Employee Number:</label>
- <input type="text" id="emp_no" name="emp_no">
- <input type="submit" value="Search">
- </form>
- <?php
- // Check if form is submitted
- if ($_SERVER["REQUEST_METHOD"] == "GET" && isset($_GET['emp_no'])) {
- $emp_no = $_GET['emp_no'];
- // Connect to MySQL database
- $servername = "localhost";
- $username = "your_username";
- $password = "your_password";
- $dbname = "your_database_name";
- $conn = new mysqli($servername, $username, $password, $dbname);
- // Check connection
- if ($conn->connect_error) {
- die("Connection failed: " . $conn->connect_error);
- }
- // Prepare SQL query
- $sql = "SELECT * FROM employees WHERE emp_no = ?";
- $stmt = $conn->prepare($sql);
- $stmt->bind_param("i", $emp_no);
- // Execute query
- $stmt->execute();
- $result = $stmt->get_result();
- // Display results in a table
- if ($result->num_rows > 0) {
- echo "<h3>Search Results:</h3>";
- echo "<table>";
- echo "<tr><th>Employee Number</th><th>Employee Name</th><th>Designation</th><th>Date of Birth</th><th>Basic Pay</th><th>DA</th></tr>";
- while ($row = $result->fetch_assoc()) {
- echo "<tr>";
- echo "<td>" . $row['emp_no'] . "</td>";
- echo "<td>" . $row['emp_name'] . "</td>";
- echo "<td>" . $row['designation'] . "</td>";
- echo "<td>" . $row['dob'] . "</td>";
- echo "<td>" . $row['basic_pay'] . "</td>";
- echo "<td>" . $row['da'] . "</td>";
- echo "</tr>";
- }
- echo "</table>";
- } else {
- echo "<p>No employee found with Employee Number: $emp_no</p>";
- }
- // Close statement and database connection
- $stmt->close();
- $conn->close();
- }
- ?>
- </body>
- </html>