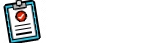
- To create a slideshow using HTML, CSS, and JavaScript, you can follow these steps:
- First, create a container element in your HTML where the slideshow will be displayed. This could be a <div> element with a specified id attribute that you can use to reference it in your CSS and JavaScript code.
- Next, create the HTML for the slides in the slideshow. Each slide should be represented by an <img> element, and all of the <img> elements should be placed inside the container element you created in step 1.
- In your CSS, you can use the #id selector to target the container element and define its dimensions, as well as the dimensions for the <img> elements. You can also use CSS to define the initial position of the slides and any other styles you want to apply to the slideshow.
- In your JavaScript, you can use the querySelector() method to access the container element and the <img> elements inside it. You can then create a function that changes the position of the slides using the style property, and create next and previous buttons that call this function when clicked.
- Finally, you can add a hyperlink to each slide by wrapping the <img> element in an <a> element and specifying the href attribute. This will allow users to click on the slides to visit the specified URL.
- Here is an example of what your HTML, CSS, and JavaScript code might look like:
- HTML:
- <div id="slideshow-container">
- <img src="slide1.jpg" alt="Slide 1">
- <img src="slide2.jpg" alt="Slide 2">
- <img src="slide3.jpg" alt="Slide 3">
- </div>
- CSS:
- #slideshow-container {
- width: 500px;
- height: 300px;
- position: relative;
- }
- #slideshow-container img {
- width: 500px;
- height: 300px;
- position: absolute;
- top: 0;
- left: 0;
- }
- JavaScript:
- <script>
- // Get the container element and the <img> elements inside it
- const container = document.querySelector('#slideshow-container');
- const slides = container.querySelectorAll('img');
- // Set the initial slide index to 0 (the first slide)
- let slideIndex = 0;
- // Function to change the slide
- function changeSlide(newIndex) {
- // Update the slide index
- slideIndex = newIndex;
- // If the new index is less than 0, set it to the last slide
- if (slideIndex < 0) {
- slideIndex = slides.length - 1;
- }
- // If the new index is greater than the last index, set it to 0 (the first slide)
- else if (slideIndex >= slides.length) {
- slideIndex = 0;
- }
- // Loop through all of the slides and set their z-index to 0
- // This will make them overlap and hide all of the slides except the current one
- for (let i = 0; i < slides.length; i++) {
- slides[i].style.zIndex = 0;
- }
- // Set the z-index of the current slide to 1, which will make it appear above the other slides
- slides[slideIndex].style.zIndex = 1;
- }
- // Create next and previous buttons
- const nextBtn = document.createElement('button');
- nextBtn.innerHTML = 'Next';
- nextBtn.addEventListener('click', () => changeSlide(slideIndex + 1));
- const prevBtn = document.createElement('button');
- prevBtn.innerHTML = 'Previous';
- prevBtn.addEventListener('click', () => changeSlide(slideIndex - 1));
- // Add the buttons to the container element
- container.appendChild(nextBtn);
- container.appendChild(prevBtn);
- // Initialize the slideshow by showing the first slide
- changeSlide(0);
- </script>
- Note that this is just one possible way to create a slideshow using HTML, CSS, and JavaScript. There are many other ways to accomplish the same thing, and you may need to adjust the code to fit your specific needs and requirements.