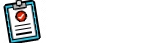
- Yes, you can use FastAPI with Django. FastAPI is a web framework for building APIs with Python, while Django is a web framework for building full-stack web applications with Python. These frameworks can be used together to create applications that have both a frontend built with Django and a backend API built with FastAPI.
- To use FastAPI with Django, you can create a FastAPI app that runs as a standalone server, and then use Django to serve the frontend and make API calls to the FastAPI server. Alternatively, you can integrate the FastAPI app into your Django project and use Django's URL routing to serve the FastAPI app alongside the Django app.
- Here is an example of how you might integrate FastAPI into a Django project:
- In your Django project, create a new directory called fastapi_app to contain your FastAPI code.
- In the fastapi_app directory, create a file called main.py with the following code to create a simple FastAPI app:
- from fastapi import FastAPI
- app = FastAPI()
- @app.get("/")
- def root():
- return {"message": "Hello, world!"}
- In your Django project's urls.py file, add the following code to include the FastAPI app in your Django project's URL routing:
- from django.contrib import admin
- from django.urls import path, include
- from fastapi_app import main
- urlpatterns = [
- path("admin/", admin.site.urls),
- path("api/", include(main.app.urls)),
- ]
- This will add the FastAPI app to your Django project at the URL /api/, so you can access the API at that URL.
- To deploy the app using Docker, you will need to do the following:
- Create a Dockerfile for the app that specifies the dependencies and commands needed to build and run the app in a Docker container.
- Create a GitHub Actions workflow file that defines the steps for building, testing, and deploying the app.
- Push the app code and the Dockerfile to a GitHub repository.
- Configure the workflow file to run on your repository, so that it automatically builds and deploys the app whenever you push changes to the repository.
- Here is an example of a Dockerfile that can be used to build and run the app in a Docker container:
- # Use a Python base image
- FROM python:3.8
- # Copy the app code into the container
- COPY . /app
- # Install the app dependencies
- RUN pip install -r /app/requirements.txt
- # Set the working directory
- WORKDIR /app
- # Run the app
- CMD ["python", "main.py"]
- This Dockerfile will use a Python base image, copy the app code into the container, install the dependencies, and run the app.
Python software and documentation are licensed under the PSF License Agreement.
Starting with Python 3.8.6, examples, recipes, and other code in the documentation are dual licensed under the PSF License Agreement and the Zero-Clause BSD license.
Some software incorporated into Python is under different licenses. The licenses are listed with code falling under that license. See Licenses and Acknowledgements for Incorporated Software for an incomplete list of these licenses.
Python and it's documentation is:
Copyright © 2001-2022 Python Software Foundation. All rights reserved.
Copyright © 2000 BeOpen.com. All rights reserved.
Copyright © 1995-2000 Corporation for National Research Initiatives. All rights reserved.
Copyright © 1991-1995 Stichting Mathematisch Centrum. All rights reserved.
See History and License for complete license and permissions information:
https://docs.python.org/3/license.html#psf-license