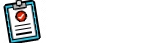
- // Base class representing a general bank account
- public class BankAccount {
- private double balance;
- // Constructor to initialize the account with a starting balance
- public BankAccount(double initialBalance) {
- this.balance = initialBalance;
- }
- // Method to deposit money into the account
- public void deposit(double amount) {
- if (amount > 0) {
- balance += amount;
- System.out.println("Deposited: $" + amount);
- } else {
- System.out.println("Deposit amount must be positive.");
- }
- }
- // Method to withdraw money from the account
- public void withdraw(double amount) {
- if (amount > 0 && amount <= balance) {
- balance -= amount;
- System.out.println("Withdrew: $" + amount);
- } else {
- System.out.println("Insufficient funds or invalid amount.");
- }
- }
- // Method to check the current balance
- public double getBalance() {
- return balance;
- }
- }
- // Subclass representing a savings account
- public class SavingsAccount extends BankAccount {
- // Constructor to initialize the savings account with a starting balance
- public SavingsAccount(double initialBalance) {
- super(initialBalance);
- }
- // Overridden withdraw method to prevent withdrawals if balance falls below $100
- @Override
- public void withdraw(double amount) {
- if (amount > 0 && (getBalance() - amount) >= 100) {
- super.withdraw(amount);
- } else {
- System.out.println("Withdrawal denied. Balance would fall below $100.");
- }
- }
- }
- // Main class to test the BankAccount and SavingsAccount classes
- public class Main {
- public static void main(String[] args) {
- // Create a SavingsAccount with an initial balance
- SavingsAccount mySavings = new SavingsAccount(500);
- // Deposit some money
- mySavings.deposit(200);
- // Attempt to withdraw an amount
- mySavings.withdraw(550); // This should be denied as it would drop the balance below $100
- // Check the current balance
- System.out.println("Current Balance: $" + mySavings.getBalance());
- // Withdraw an allowed amount
- mySavings.withdraw(200); // This should be successful
- // Check the final balance
- System.out.println("Final Balance: $" + mySavings.getBalance());
- }
- }