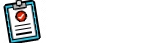
- Best ETL architecture on AWS to get large csv data files from s3 with 1000 columns and clean the data, and filter the data to only include a set of 30 columns, and then import the data into a postgres database. Show a solution using pandas or pyspark.
- There are a few different approaches you can take to ETL (extract, transform, and load) large CSV data files from Amazon S3 and import them into a PostgreSQL database using AWS services. Here is one potential solution using either Pandas or PySpark:
- Extract the data from S3: You can use the boto3 library to connect to S3 and read the data directly into a Pandas dataframe or a Spark DataFrame. For example:
- import boto3
- # Connect to S3 and read the data into a Pandas dataframe
- s3 = boto3.client('s3')
- obj = s3.get_object(Bucket='my-bucket', Key='data.csv')
- df = pd.read_csv(obj['Body'])
- # OR, if using PySpark:
- spark = pyspark.sql.SparkSession.builder.getOrCreate()
- df = spark.read.csv('s3://my-bucket/data.csv', header=True)
- Clean and filter the data: Once you have the data in a Pandas or Spark DataFrame, you can use the various data manipulation and transformation functions to clean and filter the data as needed. For example:
- # Drop any rows with null values
- df = df.dropna()
- # Select only the columns we want to keep
- df = df[['col1', 'col2', 'col3', ...]]
- # Perform any other transformations or cleaning steps as needed
- Load the data into PostgreSQL: To load the data into a PostgreSQL database, you can use the psycopg2 library to connect to the database and write the data directly from the Pandas or Spark DataFrame. For example:
- import psycopg2
- # Connect to the database
- conn = psycopg2.connect(host='my-database.abc123.us-east-1.rds.amazonaws.com',
- user='myuser', password='mypassword',
- dbname='mydatabase')
- # Write the data to the database
- df.to_sql('table_name', conn, if_exists='replace')
- # OR, if using PySpark:
- df.write.jdbc(url='jdbc:postgresql://my-database.abc123.us-east-1.rds.amazonaws.com/mydatabase',
- table='table_name', mode='overwrite', properties={'user': 'myuser', 'password': 'mypassword'})
- # Close the connection
- conn.close()
- This is just one example of an ETL pipeline for handling large CSV data files on AWS. There are many other approaches you could take depending on your specific needs and constraints.