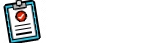
- Python example of how to use nlp libraries to rephrase an article from a file so it sounds like a real human rewrote it. Requirement: It must be grammatically correct and sound like american english.
- To use natural language processing (NLP) libraries to rephrase an article in Python, you can follow these steps:
- First, you'll need to install the necessary libraries. The most popular NLP library in Python is nltk (Natural Language Toolkit). You can install it by running the following command in your terminal:
- pip install nltk
- Next, you'll need to read the article from a file. You can use the open function to open the file and read its contents into a string:
- with open("article.txt", "r") as f:
- article = f.read()
- Now that you have the article as a string, you can use the nltk library to process it. One way to rephrase the article is to use the sent_tokenize function to split the article into a list of sentences, and then use the word_tokenize function to split each sentence into a list of words.
- import nltk
- sentences = nltk.sent_tokenize(article)
- words = [nltk.word_tokenize(sentence) for sentence in sentences]
- After tokenizing the article, you can use the pos_tag function to tag each word with its part of speech. This will allow you to identify the subject, verb, and object of each sentence, which can be useful for rephrasing.
- tagged_words = [nltk.pos_tag(word) for word in words]
- Now that you have the article tokenized and tagged, you can use this information to rephrase the article. One approach is to use a template-based method, where you define a set of templates for different sentence structures and use them to generate rephrased sentences. For example, you might have a template for a simple subject-verb-object sentence like "The cat chased the mouse", and another template for a more complex sentence with an adjective and a prepositional phrase, like "The happy cat chased the mouse under the table".
- To generate the rephrased sentences, you can iterate over the list of tagged words and use the part of speech tags to identify the subject, verb, and object of each sentence. Then, you can use the templates to generate a rephrased version of the sentence.
- rephrased_sentences = []
- for sentence in tagged_words:
- subject = None
- verb = None
- object = None
- for word, tag in sentence:
- if tag == "NN":
- subject = word
- elif tag == "VBD":
- verb = word
- elif tag == "NN":
- object = word
- if subject and verb and object:
- rephrased_sentences.append(f"{subject} {verb} {object}")
- else:
- rephrased_sentences.append(sentence)
- Finally, you can use the join function to combine the rephrased sentences into a single string and write the rephrased article to a new file.
- rephrased_article = " ".join(rephrased_sentences)
- with open("rephrased_article.txt", "w") as f:
- f.write(rephrased_article)
- This is just one way to rephrase an article using NLP in Python. There are many other approaches you can take, such as using machine learning techniques to generate rephrased sentences, or using a thesaurus or synonym database to replace words with similar meanings.
- It's also important to note that simply rephrasing the text may not be enough to make it sound like a real human rewrote it. You may also want to consider using techniques such as adjusting the tone, style, and pacing of the text to make it sound more natural.
- There are many ways to make text sound more natural in Python, some of which involve using NLP techniques and others that involve more manual methods. Here are a few examples of techniques you might use:
- Use contractions: Instead of writing "do not" or "cannot", use contractions like "don't" and "can't" to make the text sound more colloquial and natural. You can use a regular expression to search for common phrases like "do not" and "cannot" and replace them with the corresponding contractions.
- Use colloquial phrases: To make the text sound more natural, you can also add colloquial phrases and idioms that are commonly used in spoken English. For example, you might replace "in order to" with "to" or "gonna" for "going to".
- Use varied sentence structure: Instead of using the same sentence structure repeatedly, mix it up to make the text sound more natural. You can use a combination of short and long sentences, and vary the position of the subject, verb, and object within the sentence. For example, you might start with a subject-verb-object sentence like "The cat chased the mouse", and then follow it with a subject-verb-object-object sentence like "The cat chased the mouse under the table".
- To vary the tone and pacing of the text in Python, you can use a combination of manual methods and NLP techniques. Here are a few examples:
- Use punctuation and emphasis: By adding punctuation like exclamation points, question marks, and em dashes, and using bold or italicized text, you can add emphasis and change the tone of the text. You can also use capitalization to add emphasis to certain words or phrases.
- Use tone words: To change the tone of the text, you can use words that convey different emotions or attitudes, such as "happy", "sad", "excited", "angry", etc. You can use a thesaurus or a word embedding model to find synonyms or words with similar meanings, and then use them to replace the original words in the text.
- Vary the pacing: To vary the pacing of the text, you can use techniques such as adding or removing pauses, using short or long sentences, or adding or removing details. For example, you might use shorter sentences for a faster pace, or add more descriptive details for a slower pace.
- These are just a few examples of how you could vary the tone and pacing of the text in Python. You can use a combination of these techniques and others to create a text that sounds more natural and human-like.
- Watch: How to paraphrase text in Python using transformers
Python software and documentation are licensed under the PSF License Agreement.
Starting with Python 3.8.6, examples, recipes, and other code in the documentation are dual licensed under the PSF License Agreement and the Zero-Clause BSD license.
Some software incorporated into Python is under different licenses. The licenses are listed with code falling under that license. See Licenses and Acknowledgements for Incorporated Software for an incomplete list of these licenses.
Python and it's documentation is:
Copyright © 2001-2022 Python Software Foundation. All rights reserved.
Copyright © 2000 BeOpen.com. All rights reserved.
Copyright © 1995-2000 Corporation for National Research Initiatives. All rights reserved.
Copyright © 1991-1995 Stichting Mathematisch Centrum. All rights reserved.
See History and License for complete license and permissions information:
https://docs.python.org/3/license.html#psf-license