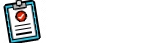
- Here is a sample Python script that demonstrates how to perform CRUD (create, read, update, and delete) operations on a PostgreSQL database, including how to perform complex joins:
- import psycopg2
- # Connect to the database
- conn = psycopg2.connect(
- host="localhost",
- port=5432,
- user="myuser",
- password="mypassword",
- dbname="mydatabase"
- )
- # Create a cursor
- cur = conn.cursor()
- # CREATE
- # Insert a new row into the table
- cur.execute("INSERT INTO users (username, password) VALUES (%s, %s)", ("john", "password123"))
- # COMMIT the changes to the database
- conn.commit()
- # READ
- # Select all rows from the table
- cur.execute("SELECT * FROM users")
- # Fetch the results
- rows = cur.fetchall()
- # Print the rows
- for row in rows:
- print(row)
- # UPDATE
- # Update the password for the user with the username "john"
- cur.execute("UPDATE users SET password = %s WHERE username = %s", ("newpassword456", "john"))
- # COMMIT the changes to the database
- conn.commit()
- # DELETE
- # Delete the user with the username "john"
- cur.execute("DELETE FROM users WHERE username = %s", ("john",))
- # COMMIT the changes to the database
- conn.commit()
- # Close the cursor and connection
- cur.close()
- conn.close()
- This script first connects to the PostgreSQL database using the psycopg2 library. It then creates a cursor, which allows you to execute SQL statements.
- The script then demonstrates how to perform each of the CRUD operations:
- CREATE: Insert a new row into the users table using the INSERT statement.
- READ: Select all rows from the users table using the SELECT statement, and print the rows.
- UPDATE: Update the password for the user with the username "john" using the UPDATE statement.
- DELETE: Delete the user with the username "john" using the DELETE statement.
- Finally, the script closes the cursor and connection to the database.
- To perform complex joins in this script, you can use the JOIN clause in your SELECT, UPDATE, or DELETE statements. For example, to select all rows from the users table and their corresponding rows in the orders table, you could use a JOIN clause like this:
- cur.execute("SELECT * FROM users INNER JOIN orders ON users.id = orders.user_id")
- This will select all rows from the users and orders tables where the id column in the users table matches the user_id column in the orders table. You can use other types of joins (e.g. LEFT JOIN, RIGHT JOIN, FULL OUTER JOIN) and specify conditions in the ON clause to further filter the results of the join.
Python software and documentation are licensed under the PSF License Agreement.
Starting with Python 3.8.6, examples, recipes, and other code in the documentation are dual licensed under the PSF License Agreement and the Zero-Clause BSD license.
Some software incorporated into Python is under different licenses. The licenses are listed with code falling under that license. See Licenses and Acknowledgements for Incorporated Software for an incomplete list of these licenses.
Python and it's documentation is:
Copyright © 2001-2022 Python Software Foundation. All rights reserved.
Copyright © 2000 BeOpen.com. All rights reserved.
Copyright © 1995-2000 Corporation for National Research Initiatives. All rights reserved.
Copyright © 1991-1995 Stichting Mathematisch Centrum. All rights reserved.
See History and License for complete license and permissions information:
https://docs.python.org/3/license.html#psf-license