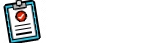
- How can I use Python's advanced object-oriented features, such as metaclasses and decorators, to design a more flexible and reusable codebase?
- Metaclasses and decorators are advanced object-oriented features in Python that can be used to modify the behavior of classes and methods in a flexible and reusable way. Here is a simple example of how you might use these features to design a more flexible and reusable codebase in Python:
- import functools
- def cache(func):
- """A decorator that caches the return value of a function."""
- @functools.wraps(func)
- def wrapper(*args, **kwargs):
- key = (args, frozenset(kwargs.items()))
- if key not in wrapper.cache:
- wrapper.cache[key] = func(*args, **kwargs)
- return wrapper.cache[key]
- wrapper.cache = {}
- return wrapper
- class CachedMeta(type):
- """A metaclass that applies the cache decorator to all methods."""
- def __new__(cls, name, bases, namespace):
- for attr, value in namespace.items():
- if callable(value):
- namespace[attr] = cache(value)
- return type.__new__(cls, name, bases, namespace)
- class MyClass(metaclass=CachedMeta):
- def slow_method(self, arg):
- # Some slow computation here
- return result
- In this example, the cache decorator is used to cache the return value of a function so that it only needs to be computed once. The CachedMeta metaclass is then used to apply this decorator to all methods in a class, so that all of their return values are automatically cached. This can be a useful way to improve the performance of a class that has many slow methods, without having to manually apply the decorator to each one.
- Note that this is just a simple example, and there are many other ways that metaclasses and decorators can be used to design a more flexible and reusable codebase in Python. For more information and examples, you may want to consult the Python documentation or other resources on these advanced object-oriented features.
Python software and documentation are licensed under the PSF License Agreement.
Starting with Python 3.8.6, examples, recipes, and other code in the documentation are dual licensed under the PSF License Agreement and the Zero-Clause BSD license.
Some software incorporated into Python is under different licenses. The licenses are listed with code falling under that license. See Licenses and Acknowledgements for Incorporated Software for an incomplete list of these licenses.
Python and it's documentation is:
Copyright © 2001-2022 Python Software Foundation. All rights reserved.
Copyright © 2000 BeOpen.com. All rights reserved.
Copyright © 1995-2000 Corporation for National Research Initiatives. All rights reserved.
Copyright © 1991-1995 Stichting Mathematisch Centrum. All rights reserved.
See History and License for complete license and permissions information:
https://docs.python.org/3/license.html#psf-license