- #include <iostream>
- class Time {
- private:
- int hour, minute, second;
- public:
- // Member function to read time
- void readTime() {
- std::cout << "Enter time (hh mm ss): ";
- std::cin >> hour >> minute >> second;
- }
- // Member function to display time
- void displayTime() {
- std::cout << "Time: " << hour << ":" << minute << ":" << second << std::endl;
- }
- // Overloading '+' operator to find the sum of two time objects
- Time operator+(const Time& t) const {
- Time sum;
- sum.second = (second + t.second) % 60;
- sum.minute = (minute + t.minute + (second + t.second) / 60) % 60;
- sum.hour = (hour + t.hour + (minute + t.minute + (second + t.second) / 60) / 60) % 24;
- return sum;
- }
- };
- int main() {
- Time time1, time2, sum;
- // Reading two times
- time1.readTime();
- time2.readTime();
- // Displaying the entered times
- std::cout << "\nEntered Times:\n";
- time1.displayTime();
- time2.displayTime();
- // Finding the sum of two times using overloaded '+' operator
- sum = time1 + time2;
- // Displaying the sum of times
- std::cout << "\nSum of Times:\n";
- sum.displayTime();
- return 0;
- }
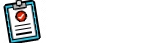